Add Two Numbers - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Example 1 :
Input: l1 = [2,4,3], l2 = [5,6,4]
Output: [7,0,8]
Explanation: 342 + 465 = 807.
Example 3 :
Input: l1 = [9,9,9,9,9,9,9], l2 = [9,9,9,9]
Output: [8,9,9,9,0,0,0,1]
배열로 보이지만 문제를 잘 읽어보면 linked list의 문제이다.
sum이라는 변수에 while문을 돌려 해당 value값을 차례로 담아준다.
그리고 생성해 놓았던 current.next ListNode 생성
이 때 이항연산자 나머지(%)를 사용하여 값을 반환하고 그 값을 ListNode(sum)에 넣어준다.
그 값을 current에 또 담아주고 삼항연산자를 이용하여 sum이 9보다 작으면 0, 크면 1을 반환한다.
My Wrong Answer :
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} l1
* @param {ListNode} l2
* @return {ListNode}
*/
const addTwoNumbers = (l1, l2) => {
let sum = 0;
// listnode 생성
let current = new ListNode(0);
// 첫번째 주소 기억(참조하기 위해)
let result = current;
while(l1 || l2) {
if(l1) {
//1. 2
//2. 4
//3. 1+3
//9
//1+9
//1+9
//1+9
//1+9
//1+9
//1+9
sum += l1.val;
//1. 4,3 최초 while allocation
//999999
l1 = l1.next
}
if(l2) {
//1. 2+5
//2. 4+6
//3. 4+4
//9+9
//10+9
//10+9
//10+9
//10+0
//10+0
//10+0
sum += l2.val;
//1. 6,4 최초 while allocation
//999
l2 = l2.next;
}
//1. ListNode(7) [0,7]
//2. ListNode(0) [0,7,1]
//3. ListNode(8) [0,7,8]
// ListNode(8) [0,8]
// ListNode(9) [0,8,9]
// ListNode(9) [0,8,9,9]
// ListNode(9) [0,8,9,9,9]
// ListNode(0) [0,8,9,9,9,0]
// ListNode(0) [0,8,9,9,9,0,0]
// ListNode(0) [0,8,9,9,9,0,0,0]
current.next = new ListNode(sum % 10);
//1. 7
//2. 10
//3. 8
current = current.next;
//1. 7 = sum에 0 할당
//2. 10 = sum에 1 할당
//3. 8 = sum에 0 할당
// 18=1
// 19=1
// 19=1
// 19=1
// 10=1
// 10=1
// 10=1
sum = sum > 9 ? 1 : 0;
}
return result.next
};
처음 작성한 코드로 실행하게 되면 example3에서 output 마지막 1이 붙지 않기 때문에 오류가 난다.
이에 삼항연산자로 인해 반환되는 값으로 if문을 사용해서 current.next에 생성해준다.
여기서 생성이 되더라도 while문이 실행될 때 재차 ListNode값이 담기는 구간이 있으므로 값은 덮어씌워지며
마지막 구문에서 1이 붙으며 해당 함수는 끝이난다.
My Answer :
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} l1
* @param {ListNode} l2
* @return {ListNode}
*/
const addTwoNumbers = (l1, l2) => {
let sum = 0;
// listnode 생성
let current = new ListNode(0);
// 첫번째 주소 기억(참조하기 위해)
let result = current;
while(l1 || l2) {
if(l1) {
//1. 2
//2. 4
//3. 1+3
//9
//1+9
//1+9
//1+9
//1+9
//1+9
//1+9
sum += l1.val;
//1. 4,3 최초 while allocation
//999999
l1 = l1.next
}
if(l2) {
//1. 2+5
//2. 4+6
//3. 4+4
//9+9
//10+9
//10+9
//10+9
//10+0
//10+0
//10+0
sum += l2.val;
//1. 6,4 최초 while allocation
//999
l2 = l2.next;
}
//1. ListNode(7) [0,7]
//2. ListNode(0) [0,7,1]
//3. ListNode(8) [0,7,8]
// ListNode(8) [0,8]
// ListNode(9) [0,8,9]
// ListNode(9) [0,8,9,9]
// ListNode(9) [0,8,9,9,9]
// ListNode(0) [0,8,9,9,9,0]
// ListNode(0) [0,8,9,9,9,0,0]
// ListNode(0) [0,8,9,9,9,0,0,0]
current.next = new ListNode(sum % 10);
//1. 7
//2. 10
//3. 8
current = current.next;
//1. 7 = sum에 0 할당
//2. 10 = sum에 1 할당
//3. 8 = sum에 0 할당
// 18=1
// 19=1
// 19=1
// 19=1
// 10=1
// 10=1
// 10=1
sum = sum > 9 ? 1 : 0;
// 0. [0]
if(sum) {
//0. [0,1]
//[0,8,1]
//[0,8,9,1]
//[0,8,9,9,1]
//[0,8,9,9,9,1]
//[0,8,9,9,9,0,1]
//[0,8,9,9,9,0,0,1]
//[0,8,9,9,9,0,0,0,1]
current.next = new ListNode(sum);
}
}
return result.next
};
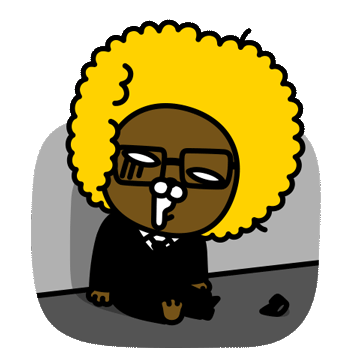
멀었다 멀었어 많이 멀었어 한~~참 멀었어....
틀린 부분이 있으시거나 더 좋은 방법이 있다면 둥글게둥글게 살포시 댓글로 알려주세요 :)
상처 잘 받는 그런 아이랍니다...
'개발공부 > Javascript' 카테고리의 다른 글
#leetcode - Two Sum (0) | 2022.01.27 |
---|
댓글